Using ASP.NET Session State in a Web Service:-
To use asp.net session object in a web service, the web service class must inherit from System.Web.Services.WebService class and EnableSession property of WebMethod attribute must be set to true.
Step(1):-Create a Web Service name is CalculateWebServices and copy and paste the following code as shown in below.
Step(2):-Create the client application named is CalculateWebServiceClient in ASP.NET Website and add a Default.aspx page.
Step(3):-Now, copy and paste the following code in Default.aspx page.
Step(4):-Copy and paste the following the code in Default.aspx.cs
Step(5):- In web.config file of CalculateWebServices, set allowCookies attribute to true.
Step(7):-Now, run the application and get output as our requirement.
Step(1):-Create a Web Service name is CalculateWebServices and copy and paste the following code as shown in below.
[WebService(Namespace = "http://santosh-asp.com/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] [System.ComponentModel.ToolboxItem(false)] public class Service1 : System.Web.Services.WebService { [WebMethod(EnableSession = true)] public int Add(int firstNumber, int secondNumber) { List calculations; if (Session["CALCULATIONS"] == null) { calculations = new List (); } else { calculations = (List )Session["CALCULATIONS"]; } string strTransaction = firstNumber.ToString() + " + " + secondNumber.ToString() + " = " + (firstNumber + secondNumber).ToString(); calculations.Add(strTransaction); Session["CALCULATIONS"] = calculations; return firstNumber + secondNumber; } [WebMethod(EnableSession = true)] public List GetCalculations() { if (Session["CALCULATIONS"] == null) { List calculations = new List (); calculations.Add("You have not performed any calculations"); return calculations; } else { return (List )Session["CALCULATIONS"]; } } }
Step(2):-Create the client application named is CalculateWebServiceClient in ASP.NET Website and add a Default.aspx page.
Step(3):-Now, copy and paste the following code in Default.aspx page.
First Number |
|
Second Number |
|
Result |
|
|
|
|
Step(4):-Copy and paste the following the code in Default.aspx.cs
protected void btnAdd_Click(object sender, EventArgs e) { ServiceReference1.Service1SoapClient objService = new ServiceReference1.Service1SoapClient(); int result = objService.Add(Convert.ToInt32(txtFirstNumber.Text), Convert.ToInt32(txtSecondNumber.Text)); lblResult.Text = result.ToString(); gvCalculations.DataSource = objService.GetCalculations(); gvCalculations.DataBind(); gvCalculations.HeaderRow.Cells[0].Text = "Recent Calculations"; }
Step(5):- In web.config file of CalculateWebServices, set allowCookies attribute to true.
Step(7):-Now, run the application and get output as our requirement.
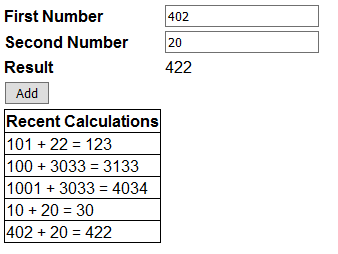