Real time example of stack collection class in c#:-
Two common scenarios, where a stack can be used.
1. Implementing UNDO functionality
2. Implementing browser back button
In this video, let's implement BACK button using stack.
Step 1: Create an asp.net web application. Use "WebFormsDemo" as the project name.
Step 2: Right click on the project name in solution explorer, and a class file with name ="BasePage.cs". Copy and paste the following code.
Step 3: Right click on the project name in solution explorer, and a master page with name = Site.Master. Copy and paste the following code.
Step 4: Copy and paste the following code in Site.Master.cs
Now click on the back button, on the page(Not the browser back button), and notice that we are able to navigate back.
1. Implementing UNDO functionality
2. Implementing browser back button
In this video, let's implement BACK button using stack.
Step 1: Create an asp.net web application. Use "WebFormsDemo" as the project name.
Step 2: Right click on the project name in solution explorer, and a class file with name ="BasePage.cs". Copy and paste the following code.
using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace WebFormsDemo { public class BasePage : System.Web.UI.Page { protected override void OnLoad(EventArgs e) { if (Session["URLStack"] == null) { Stack urlStack = new Stack (); Session["URLStack"] = urlStack; } if (Request.UrlReferrer != null && !this.Page.IsPostBack && Session["BackButtonClicked"] == null) { Stack urlStack = (Stack )Session["URLStack"]; urlStack.Push(Request.UrlReferrer.AbsoluteUri); } if (Session["BackButtonClicked"] != null) { Session["BackButtonClicked"] = null; } } } }
Step 3: Right click on the project name in solution explorer, and a master page with name = Site.Master. Copy and paste the following code.
Step 4: Copy and paste the following code in Site.Master.cs
public partial class Site : System.Web.UI.MasterPage { protected void Page_Load(object sender, EventArgs e) { } protected void btnBack_Click(object sender, EventArgs e) { Session["BackButtonClicked"] = "YES"; if (Session["URLStack"] != null) { Stack urlStack = (Stack )Session["URLStack"]; if (urlStack.Count > 0) { string url = urlStack.Pop(); Response.Redirect(url); } else { lblMessage.Text = "There are no pages in the history"; } } } }
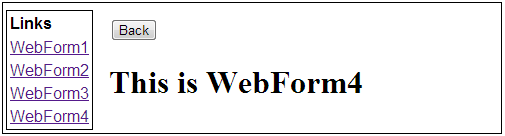
Now click on the back button, on the page(Not the browser back button), and notice that we are able to navigate back.