Create Gridview at runtime
In Asp.Net one of the most frequently used Web Control is the GridView control. In the previous examples we access data from database using SqlDataSource. So no need of Code behind use to retrieve the data. Also we fixes database , sql and everything before we run the program.
In this article we'll look at how to use the GridView to display data. In order to display data on runtime, simply drag and drop the DataGrid onto your ASP.NET page and rest of the things we set from the code behind. After drag and drop the gridview, your design page look like the following image :
After drag and drop the gridview on the form, we go to the code behind section and write the code for accessing data from database and display on Gridview.
Source code:- Default.aspx
Default.aspx.cs
In this article we'll look at how to use the GridView to display data. In order to display data on runtime, simply drag and drop the DataGrid onto your ASP.NET page and rest of the things we set from the code behind. After drag and drop the gridview, your design page look like the following image :
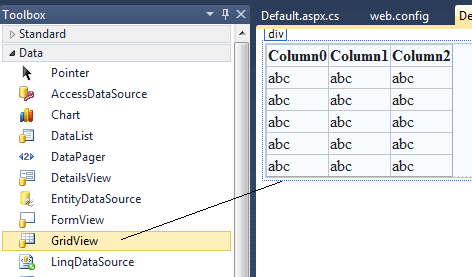
After drag and drop the gridview on the form, we go to the code behind section and write the code for accessing data from database and display on Gridview.
Source code:- Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="Default" %>
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data.SqlClient; using System.Data; public partial class Default3 : System.Web.UI.Page { string connectionString = "Data Source=PS-PC\\SANTOSH;Initial Catalog=dbSantoshTest;Integrated Security=True"; protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { loadRecord(); } } protected void loadRecord() { SqlConnection con = new SqlConnection(connectionString); con.Open(); SqlCommand cmd = new SqlCommand("Select * from tblRecord", con); SqlDataAdapter da = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); da.Fill(ds); int count = ds.Tables[0].Rows.Count; con.Close(); if (ds.Tables[0].Rows.Count > 0) { Gridview1.DataSource = ds; Gridview1.DataBind(); } else { ds.Tables[0].Rows.Add(ds.Tables[0].NewRow()); Gridview1.DataSource = ds; Gridview1.DataBind(); int columncount = Gridview1.Rows[0].Cells.Count; } } }