ASP.NET Simple GridView
The GridView control provides many built-in capabilities that allow the user to sort, update, delete, select, and page through items in the control. The GridView control can be bound to a data source control, in order to bind a data source control, set the DataSourceID property of the GridView control to the ID value of the data source control.
In this article I have used SQL SERVER database for sample data.
Before you start to generate GridView in your asp file, you should create a ConnectionString in your web.Config File. Double click the web.config file on the right hand side of the Visual Studio and add the following connectionstring code in that file.
The following is the full asp source code for selecting Id,Name,Branch from the authors table in the Pubs database.
Asp.net gridview example
Default.aspx <
When you run this asp.net application , your output look likes the following image:- 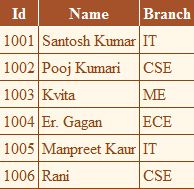
In this article I have used SQL SERVER database for sample data.
Create table tblRecord ( Id int primary key, Name varchar(200),Branch varchar(50) ) --Insert some record
Before you start to generate GridView in your asp file, you should create a ConnectionString in your web.Config File. Double click the web.config file on the right hand side of the Visual Studio and add the following connectionstring code in that file.
Web.Config File
The following is the full asp source code for selecting Id,Name,Branch from the authors table in the Pubs database.
Asp.net gridview example
Default.aspx <
When you run this asp.net application , your output look likes the following image:-