Question:136
You are developing an Azure solution.
You need to develop code to access a secret stored in Azure Key Vault.
How should you complete the code segment? To answer, drag the appropriate code segments to the correct location. Each code segment may be used once, more than once, or not at all. You may need to drag the split bar between panes or scroll to view content.
NOTE: Each correct selection is worth one point.
Select and Place:
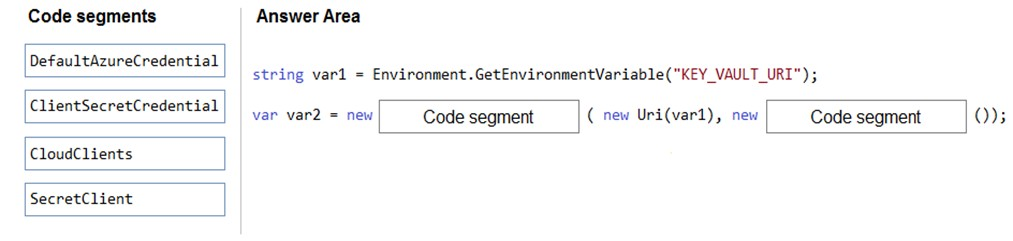
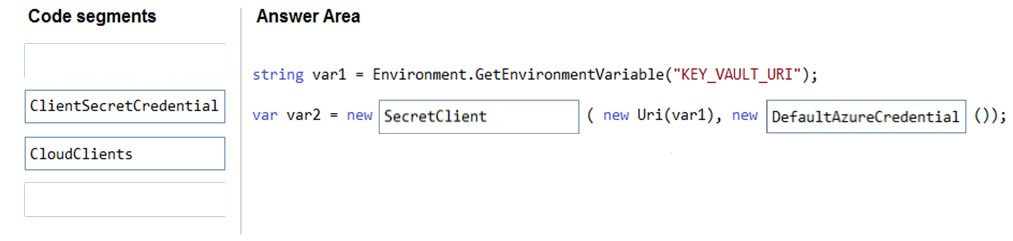
Box 1: SecretClient -
Box 2: DefaultAzureCredential -
In below example, the name of your key vault is expanded to the key vault URI, in the format "https://<your-key-vault-name>.vault.azure.net". This example is using 'DefaultAzureCredential()' class from Azure Identity Library, which allows to use the same code across different environments with different options to provide identity. string keyVaultName = Environment.GetEnvironmentVariable("KEY_VAULT_NAME"); var kvUri = "https://" + keyVaultName + ".vault.azure.net"; var client = new SecretClient(new Uri(kvUri), new DefaultAzureCredential());
https://docs.microsoft.com/en-us/azure/key-vault/secrets/quick-create-net
Question:137
You are developing an Azure App Service REST API.
The API must be called by an Azure App Service web app. The API must retrieve and update user profile information stored in Azure Active Directory (Azure AD).
You need to configure the API to make the updates.
Which two tools should you use? Each correct answer presents part of the solution.
NOTE: Each correct selection is worth one point.
- A. Microsoft Graph API
- B. Microsoft Authentication Library (MSAL)
- C. Azure API Management
- D. Microsoft Azure Security Center
- E. Microsoft Azure Key Vault SDK
A: You can use the Azure AD REST APIs in Microsoft Graph to create unique workflows between Azure AD resources and third-party services.
Enterprise developers use Microsoft Graph to integrate Azure AD identity management and other services to automate administrative workflows, such as employee onboarding (and termination), profile maintenance, license deployment, and more.
C: API Management (APIM) is a way to create consistent and modern API gateways for existing back-end services.
API Management helps organizations publish APIs to external, partner, and internal developers to unlock the potential of their data and services.
Reference:
https://docs.microsoft.com/en-us/graph/azuread-identity-access-management-concept-overview
Question:138
You develop a REST API. You implement a user delegation SAS token to communicate with Azure Blob storage.
The token is compromised.
You need to revoke the token.
What are two possible ways to achieve this goal? Each correct answer presents a complete solution.
NOTE: Each correct selection is worth one point.
- A. Revoke the delegation key.
- B. Delete the stored access policy.
- C. Regenerate the account key.
- D. Remove the role assignment for the security principle.
A: Revoke a user delegation SAS -
To revoke a user delegation SAS from the Azure CLI, call the az storage account revoke-delegation-keys command. This command revokes all of the user delegation keys associated with the specified storage account. Any shared access signatures associated with those keys are invalidated.
B: To revoke a stored access policy, you can either delete it, or rename it by changing the signed identifier. Changing the signed identifier breaks the associations between any existing signatures and the stored access policy. Deleting or renaming the stored access policy immediately effects all of the shared access signatures associated with it.
Reference:
https://github.com/MicrosoftDocs/azure-docs/blob/master/articles/storage/blobs/storage-blob-user-delegation-sas-create-cli.md https://docs.microsoft.com/en-us/rest/api/storageservices/define-stored-access-policy#modifying-or-revoking-a-stored-access-policy
Question:139
You are developing an Azure-hosted application that must use an on-premises hardware security module (HSM) key.
The key must be transferred to your existing Azure Key Vault by using the Bring Your Own Key (BYOK) process.
You need to securely transfer the key to Azure Key Vault.
Which four actions should you perform in sequence? To answer, move the appropriate actions from the list of actions to the answer area and arrange them in the correct order.
Select and Place:
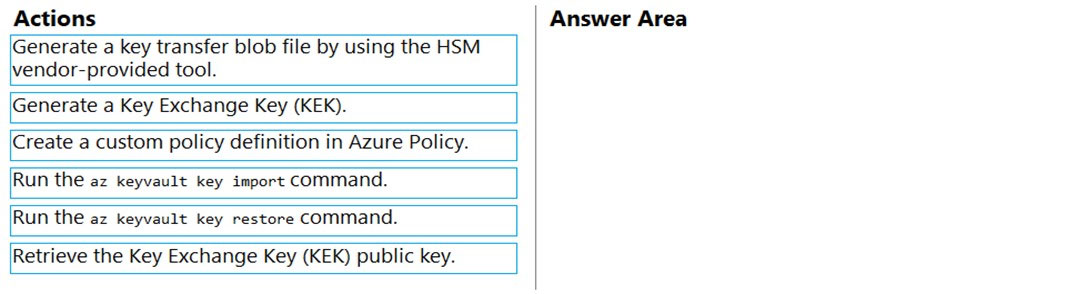
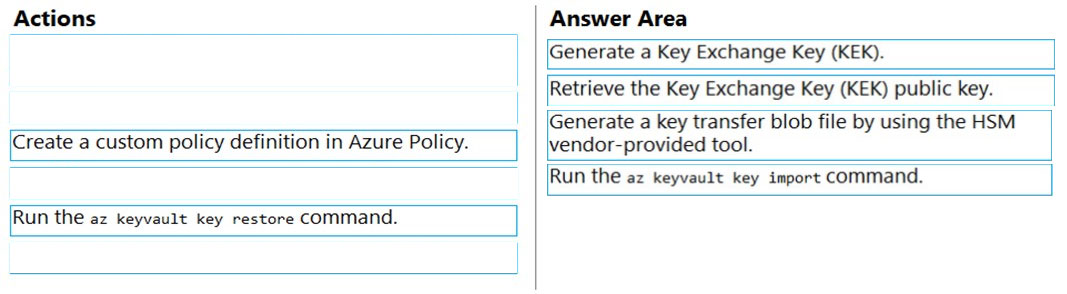
To perform a key transfer, a user performs following steps:
✑ Generate KEK.
✑ Retrieve the public key of the KEK.
✑ Using HSM vendor provided BYOK tool - Import the KEK into the target HSM and exports the Target Key protected by the KEK.
✑ Import the protected Target Key to Azure Key Vault.
Step 1: Generate a Key Exchange Key (KEK).
Step 2: Retrieve the Key Exchange Key (KEK) public key.
Step 3: Generate a key transfer blob file by using the HSM vendor-provided tool.
Generate key transfer blob using HSM vendor provided BYOK tool
Step 4: Run the az keyvault key import command
Upload key transfer blob to import HSM-key.
Customer will transfer the Key Transfer Blob (".byok" file) to an online workstation and then run a az keyvault key import command to import this blob as a new
HSM-backed key into Key Vault.
To import an RSA key use this command:
az keyvault key import
Reference:
https://docs.microsoft.com/en-us/azure/key-vault/keys/byok-specification
Question:140
You develop and deploy an Azure Logic app that calls an Azure Function app. The Azure Function app includes an OpenAPI (Swagger) definition and uses an
Azure Blob storage account. All resources are secured by using Azure Active Directory (Azure AD).
The Azure Logic app must securely access the Azure Blob storage account. Azure AD resources must remain if the Azure Logic app is deleted.
You need to secure the Azure Logic app.
What should you do?
- A. Create a user-assigned managed identity and assign role-based access controls.
- B. Create an Azure AD custom role and assign the role to the Azure Blob storage account.
- C. Create an Azure Key Vault and issue a client certificate.
- D. Create a system-assigned managed identity and issue a client certificate.
- E. Create an Azure AD custom role and assign role-based access controls.
To give a managed identity access to an Azure resource, you need to add a role to the target resource for that identity.
Note: To easily authenticate access to other resources that are protected by Azure Active Directory (Azure AD) without having to sign in and provide credentials or secrets, your logic app can use a managed identity (formerly known as Managed Service Identity or MSI). Azure manages this identity for you and helps secure your credentials because you don't have to provide or rotate secrets.
If you set up your logic app to use the system-assigned identity or a manually created, user-assigned identity, the function in your logic app can also use that same identity for authentication.
Reference:
https://docs.microsoft.com/en-us/azure/logic-apps/create-managed-service-identity https://docs.microsoft.com/en-us/azure/api-management/api-management-howto-mutual-certificates-for-clients