Insert, Update, Delete and Search by Linq
There are some steps and code for insert, update, delete and search by LINQ in SQL SERVER database in ASP.NET:-
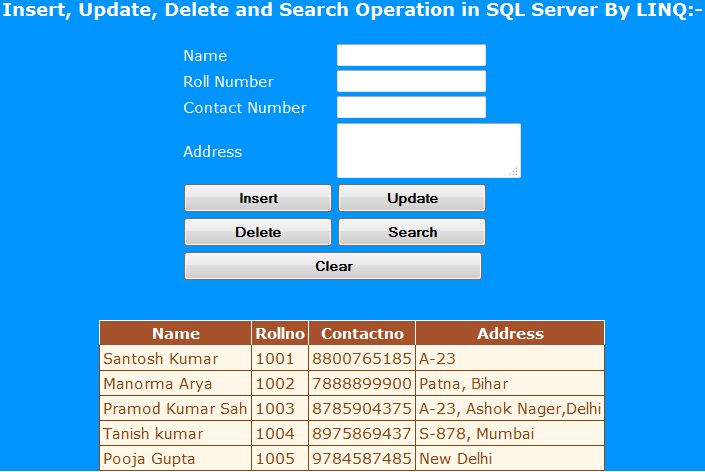
Step 1:- We are using SQL SERVER database. So, we need to create database and table in SQL SERVER as following code:-
Step 2:-
First of all we create an application and give name it LinqTest as shown in below image:-
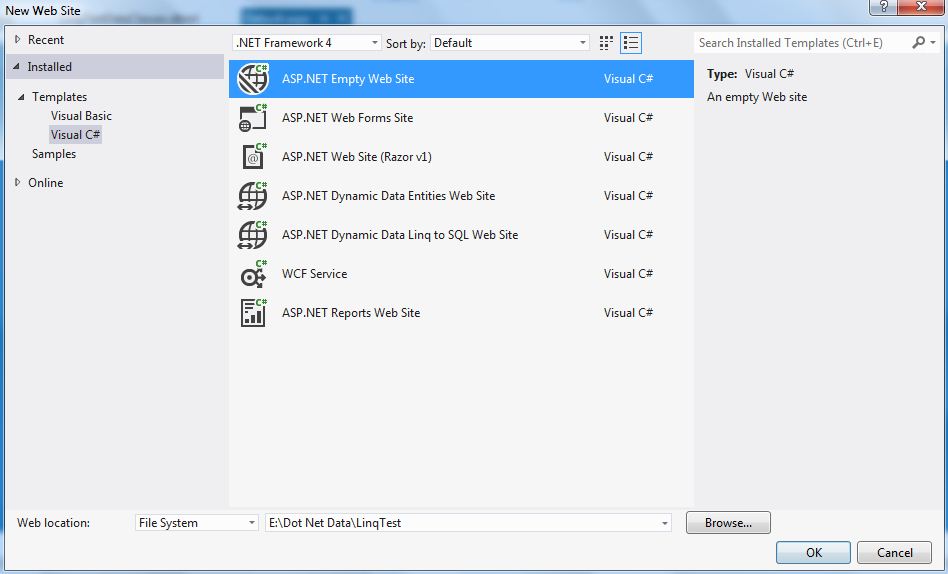
Step 3:-
Now, Go to Solution Explorer and Right click on LinqTest and go to add new item and select Webform and give name as Default.aspx page as shown in below image:-
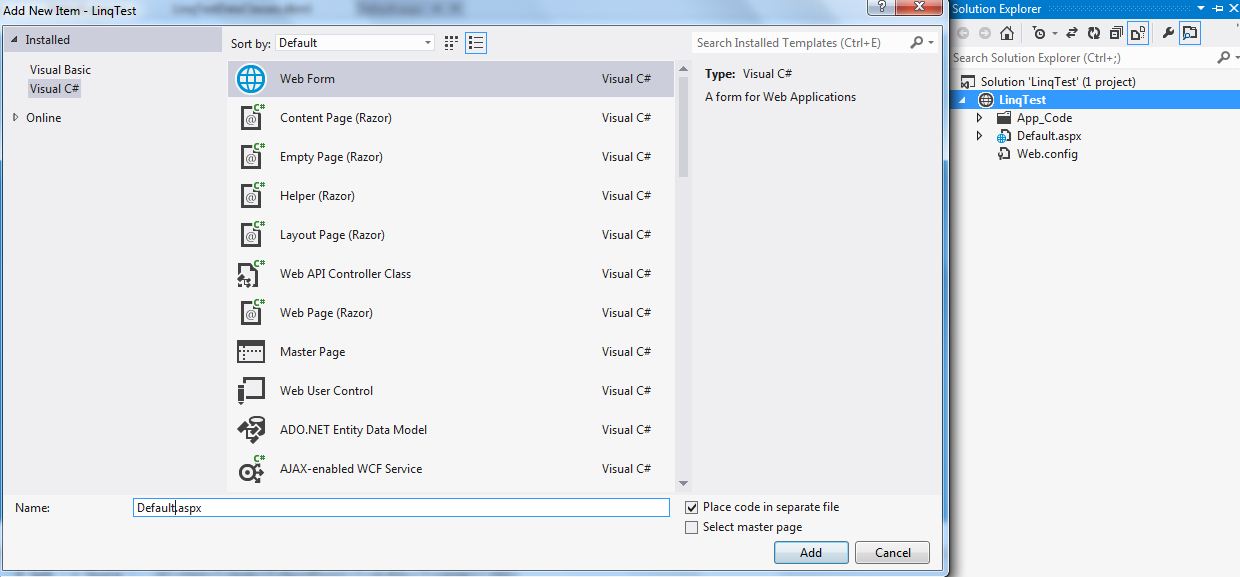
Step 4:-
Now, add following code in Default.aspx page:-
Step 5:-
Now, Go to Solution Explorer and Right click on LinqTest and go to add new item and select LINQ to SQL Classes and give name as LinqTestDataClasses.dbml as shown in below image:-
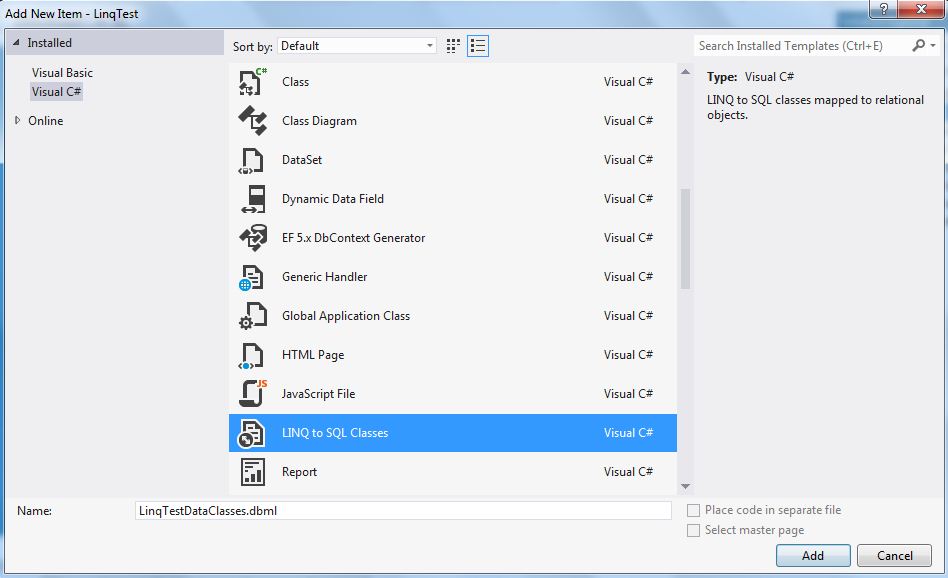
Step 6:-
Now, Go to Server Explorer and drag and drop tblLinqTest table on LinqTestDataClasses.dbml as shown in below image.
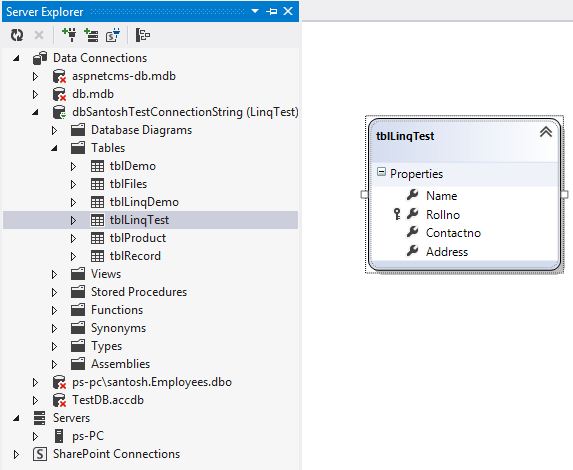
Now, our application look like this:-
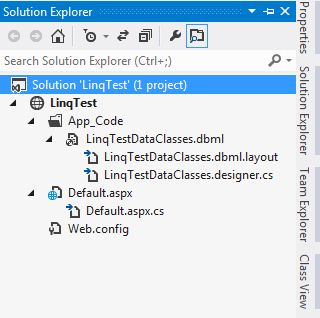
and connection string in Web.config file is as shown in below:-
Step 7:-
Now, Go to Default.aspx.cs page and add following code in our project:-
Now, run the application and Insert, Update, Delete and Search operation perform as shown in below:-
Step 1:- We are using SQL SERVER database. So, we need to create database and table in SQL SERVER as following code:-
CREATE DATABASE dbSantoshTest USE dbSantoshTest CREATE TABLE tblLinqTest ( Name varchar(200),Rollno int Primary key,Contactno bigint,Address nvarchar(max) )
Step 2:-
First of all we create an application and give name it LinqTest as shown in below image:-
Step 3:-
Now, Go to Solution Explorer and Right click on LinqTest and go to add new item and select Webform and give name as Default.aspx page as shown in below image:-
Step 4:-
Now, add following code in Default.aspx page:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
Step 5:-
Now, Go to Solution Explorer and Right click on LinqTest and go to add new item and select LINQ to SQL Classes and give name as LinqTestDataClasses.dbml as shown in below image:-
Step 6:-
Now, Go to Server Explorer and drag and drop tblLinqTest table on LinqTestDataClasses.dbml as shown in below image.
Now, our application look like this:-
and connection string in Web.config file is as shown in below:-
Step 7:-
Now, Go to Default.aspx.cs page and add following code in our project:-
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Configuration; using System.Drawing; public partial class _Default : System.Web.UI.Page { //Connection String called from Wec.config string CS = ConfigurationManager.ConnectionStrings["dbSantoshTestConnectionString"].ConnectionString; protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { LoadRecord(); } } //Bind the Gridview code private void LoadRecord() { LinqTestDataClassesDataContext objDC = new LinqTestDataClassesDataContext(); var query = from data in objDC.GetTable () select data; gvDetail.DataSource = query; gvDetail.DataBind(); } //Insert method code public void Insert() { try { LinqTestDataClassesDataContext objDC = new LinqTestDataClassesDataContext(); tblLinqTest objtblLinqTest = new tblLinqTest(); objtblLinqTest.Name = txtName.Text; objtblLinqTest.Rollno = Convert.ToInt32(txtRollno.Text); objtblLinqTest.Contactno = Convert.ToInt64(txtContactNo.Text); objtblLinqTest.Address = txtAddress.Text; objDC.tblLinqTests.InsertOnSubmit(objtblLinqTest); objDC.SubmitChanges(); LoadRecord(); Clear(); lblMessage.ForeColor = Color.Black; lblMessage.Text = "Record Is Inserted!"; } catch (Exception ex) { lblMessage.Text = ex.Message; LoadRecord(); Clear(); } } //Update method code private void Update() { try { LinqTestDataClassesDataContext objDC = new LinqTestDataClassesDataContext(); tblLinqTest objtblLinqTest = objDC.tblLinqTests.Single(tblLinqTest => tblLinqTest.Rollno == Convert.ToInt32(txtRollno.Text)); objtblLinqTest.Contactno = Convert.ToInt32(txtContactNo.Text); objtblLinqTest.Name = txtName.Text; objtblLinqTest.Address = txtAddress.Text; lblMessage.ForeColor = Color.Black; lblMessage.Text = "Record is updated!"; LoadRecord(); } catch (Exception ex) { lblMessage.Text = ex.Message; } } //Delete method code private void Delete() { try { LinqTestDataClassesDataContext objDC = new LinqTestDataClassesDataContext(); tblLinqTest objtblLinqTest = objDC.tblLinqTests.Single(tblLinqTest => tblLinqTest.Rollno == Convert.ToInt32(txtRollno.Text)); objDC.tblLinqTests.DeleteOnSubmit(objtblLinqTest); objDC.SubmitChanges(); lblMessage.ForeColor = Color.Black; lblMessage.Text = "Record is deleted!"; LoadRecord(); } catch (Exception ex) { lblMessage.Text = ex.Message; } } //Clear textbox fields code private void Clear() { txtName.Text = ""; txtRollno.Text = ""; txtContactNo.Text = ""; txtAddress.Text = ""; } protected void btnInsert_Click(object sender, EventArgs e) { Insert(); LoadRecord(); Clear(); } protected void btnUpdate_Click(object sender, EventArgs e) { Update(); LoadRecord(); Clear(); } protected void btnDelete_Click(object sender, EventArgs e) { Delete(); LoadRecord(); Clear(); } protected void btnSearch_Click(object sender, EventArgs e) { LoadRecord(); Clear(); } }
Now, run the application and Insert, Update, Delete and Search operation perform as shown in below:-