Creating a LINQ to Object Application
LINQ to Object allows you to write the queries over a collection of objects.
It refers to the use of LINQ queries with any IEnumerable or IEnumerable
interface directly.
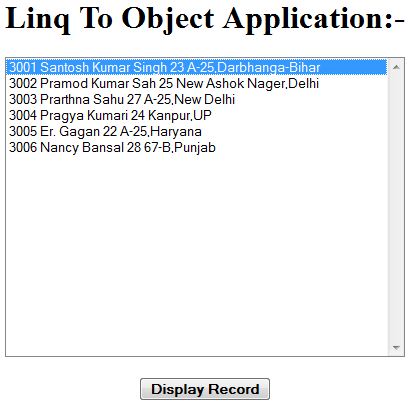
For the application we can use following step:-
Stpe 1:-
Create the empty website and give name LinqToObjectApp[11April-2015] click add button as shown in below image.
Stpe 2:-
Add Default.aspx page and write the following code in Default.aspx page:-
Stpe 3:-
Right click the application in Solution Explorer and select the Add New Item option from the context menu. This open the Add New Item dialog box. Add the class, and rename it to Employee.cs. as shown in below image.
Now your application look like as this below image.
Stpe 4:-
Add the folloing code in Employee.cs class file.
Stpe 5:-
Double click on Display Record button and write the below code:-
Now run the application and click of Display Record button and you will get output as below:-
It refers to the use of LINQ queries with any IEnumerable or IEnumerable
For the application we can use following step:-
Stpe 1:-
Create the empty website and give name LinqToObjectApp[11April-2015] click add button as shown in below image.
Stpe 2:-
Add Default.aspx page and write the following code in Default.aspx page:-
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
Stpe 3:-
Right click the application in Solution Explorer and select the Add New Item option from the context menu. This open the Add New Item dialog box. Add the class, and rename it to Employee.cs. as shown in below image.
Now your application look like as this below image.
Stpe 4:-
Add the folloing code in Employee.cs class file.
using System; using System.Collections.Generic; using System.Linq; using System.Web; /// /// Summary description for Employee /// public class Employee { public int EmpId { get; set; } public string Name { get; set; } public string Address { get; set; } public int Age { get; set; } public Employee(int empid,string name,string address,int age) { EmpId = empid; Name = name; Address = address; Age = age; } }
Stpe 5:-
Double click on Display Record button and write the below code:-
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } List GetEmployees() { List objEmp = new List (); objEmp.Add(new Employee(3001, " Santosh Kumar Singh ", " A-25,Darbhanga-Bihar ", 23)); objEmp.Add(new Employee(3002, " Pramod Kumar Sah ", " New Ashok Nager,Delhi ", 25)); objEmp.Add(new Employee(3003, " Prarthna Sahu ", " A-25,New Delhi ", 27)); objEmp.Add(new Employee(3004, "Pragya Kumari", "Kanpur,UP", 24)); objEmp.Add(new Employee(3005, "Er. Gagan ", "A-25,Haryana", 22)); objEmp.Add(new Employee(3006, "Nancy Bansal", "67-B,Punjab", 28)); return objEmp; } protected void btnDisplayRecord_Click(object sender, EventArgs e) { lbDetail.Items.Clear(); List emp = GetEmployees(); var query = from em in emp where em.Age<30 select em; foreach (var record in query) { lbDetail.Items.Add(record.EmpId + " " + record.Name + " " + record.Age + " " + record.Address); } } }
Now run the application and click of Display Record button and you will get output as below:-