Using Join clause
We can use the join clause to combine two source of data based on specific requirement:-
Now, we add the Default.aspx page and write the following code:
Now, double click on Display Record button and write the following code:-
Now, we run application and click on Display Record button and output will display according to our expectation.
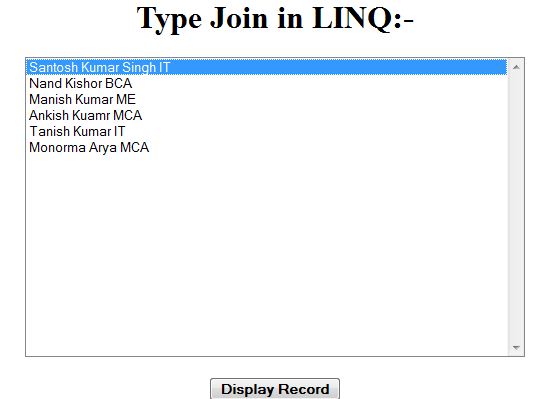
Now, we add the Default.aspx page and write the following code:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
Now, double click on Display Record button and write the following code:-
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Xml.Linq; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } public class Student { public int Rollno; public string Name; } public class Record { public int Rollno; public string Branch; } protected void btnDisplayRecord_Click(object sender, EventArgs e) { lbDetail.Items.Clear(); var students = new List () { new Student{Rollno=1001,Name="Santosh Kumar Singh"}, new Student{Rollno=1002,Name="Nand Kishor"}, new Student{Rollno=1003,Name="Manish Kumar"}, new Student{Rollno=1004,Name="Ankish Kuamr"}, new Student{Rollno=1005,Name="Tanish Kumar"}, new Student{Rollno=1006,Name="Monorma Arya"}, }; var records = new List () { new Record {Rollno=1001,Branch="IT"}, new Record {Rollno=1002,Branch="BCA"}, new Record {Rollno=1003,Branch="ME"}, new Record {Rollno=1004,Branch="MCA"}, new Record {Rollno=1005,Branch="IT"}, new Record {Rollno=1006,Branch="MCA"}, new Record {Rollno=1007,Branch="ECE"}, }; var query = from s in students join r in records on s.Rollno equals r.Rollno select new { s.Name, r.Branch }; foreach (var data in query) { lbDetail.Items.Add(data.Name.ToString() + " " + data.Branch.ToString()); } } }
Now, we run application and click on Display Record button and output will display according to our expectation.