First step in securing the application is only authenticated users are allowed to view the specific url with in the application. In MVC application you can implement this feature by using ‘Authorize’ attribute.
MVC application uses the routing engine to map the URL to the controller action. So when user sent the request through url, action method inside the controller will send the response back. Using ‘Authorize’ attribute on the action method, you can restrict the unauthorized user to get response from the system.
When ‘Authorize’ method are placed on controller or controller action, User can able to navigate to those view only by logging into the application. Application will allow only authenticated user to call these controller action.
Sample code:
MVC allows you to set the Role or User based security to the application by adding Role/User property to the‘Authorize’ attribute
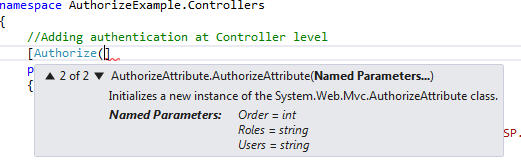
Example:-
For huge application it is always good idea to apply ‘Authorize’ at global level and allow anonymous access to specific controller. This can be achieved by registering attribute in the global filer.
File: …\...\App_Start\FilterConfig.cs
Code:
Let’s understand more about Authorize by using the default internet template MVC application.
Step 1:
Create a sample internet application from Visual Studio 2012
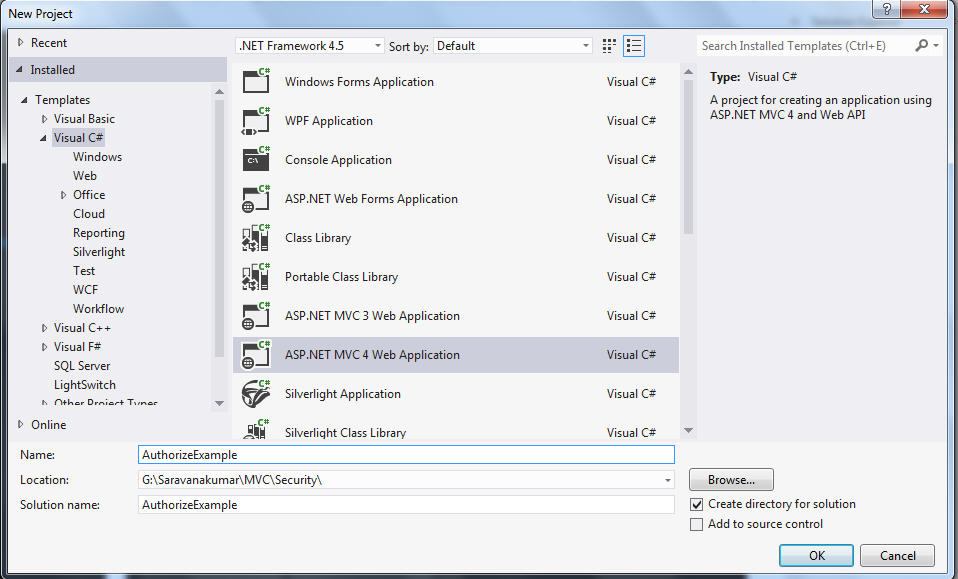
Select “Internet” application from project template and click OK.
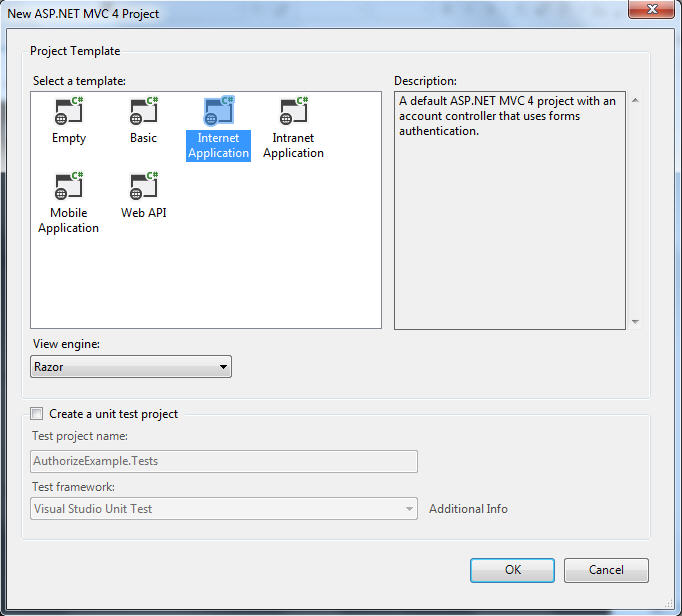
Step 2: Run the application and try to Navigate to Home, About, Contact page
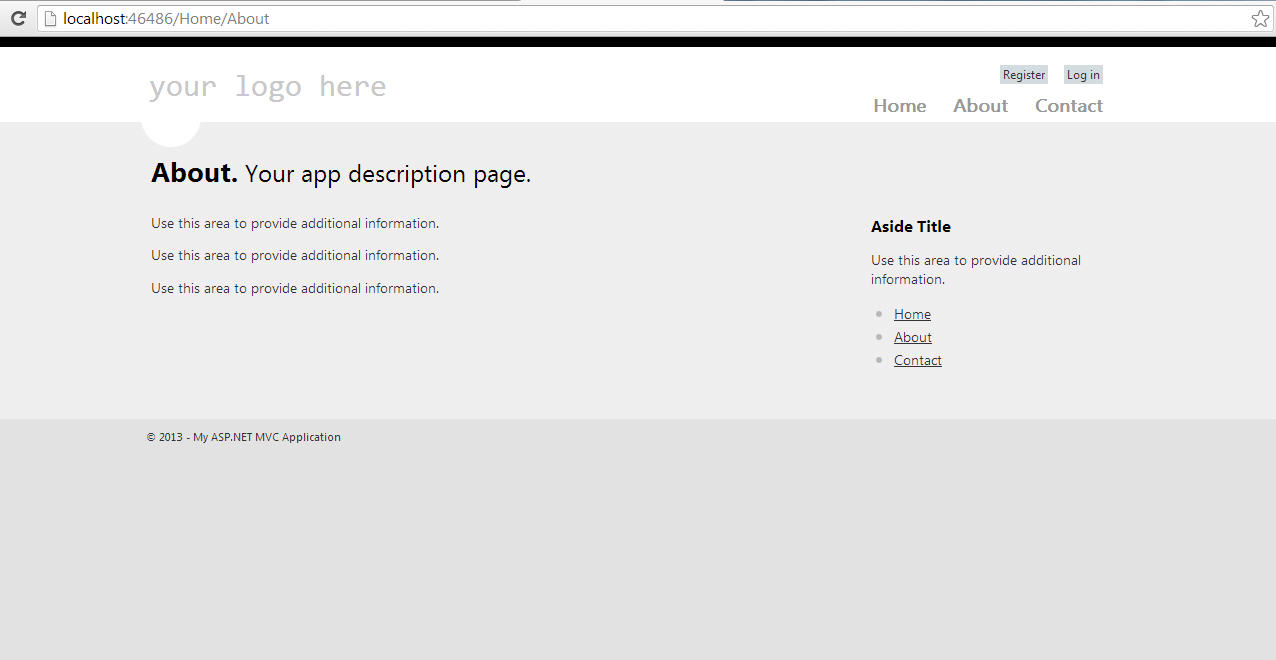
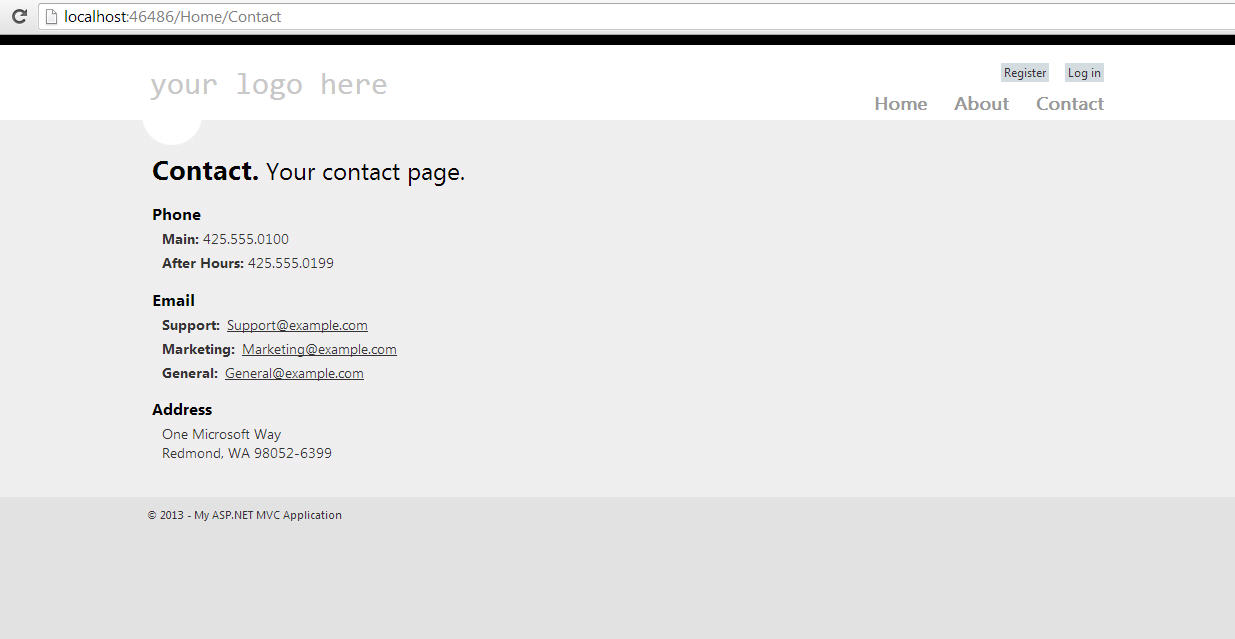
Step 3:
Now you add an [Authorize] attribute to the “About” and “Contact” action method in Home controller
Step 4:
Run the application and try to navigate to the “About” and “Contact” page, application will not allow you to view the screen. Instead it will navigate to the login screen, you have to login to web site to view the About and ‘Contact’ page. ‘Authorize’ attribute allows only authenticated user to view the screen.
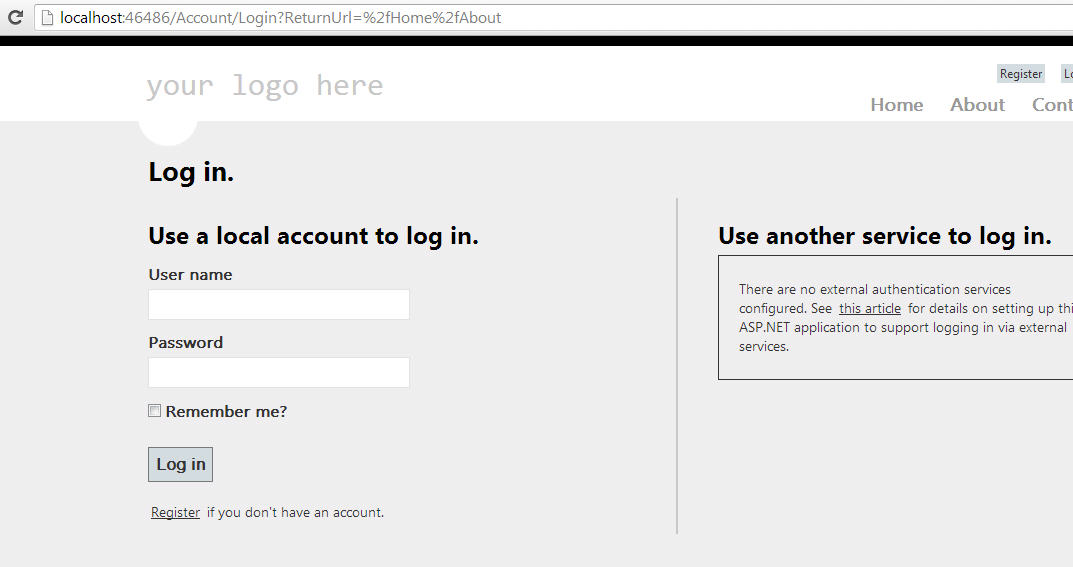
Step 5:
Let’s Register the user and view
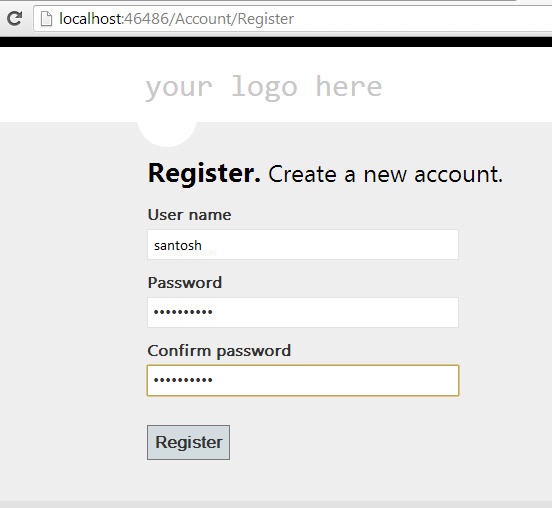
Step 6:
Application will allow you to view the About and Contact page, with registered user name.
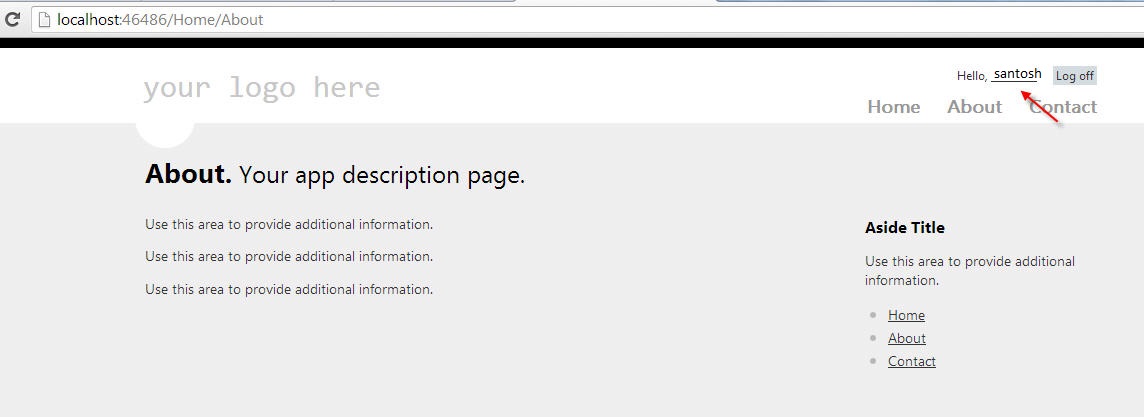