Scaffolding in MVC:-
- Scaffolding is a technique used by many MVC frameworks like ASP.NET MVC, Ruby on Rails, Cake PHP and Node.JS etc., to generate code for basic CRUD (create, read, update, and delete) operations against your database effectively. Further you can edit or customize this auto generated code according to your need.
- Scaffolding consists of page templates, entity page templates, field page templates, and filter templates. These templates are called Scaffold templates and allow you to quickly build a functional data-driven Website.
How Scaffold templates works in ASP.NET MVC:-
Scaffold templates are used to generate code for basic CRUD operations within your ASP.NET MVC applications against your database with the help Entity Framework.
These templates use the Visual Studio T4 templating system to generate views for basic CRUD operations with the help of Entity Framework.
Example:- Let’s understand about scaffolding by creating simple MVC application with edit, create, delete functionality for ‘Employee’ model object and storing the data into DB using Entity Framework.
Step 1: Create a sample MVC application with Internet project template File->New->Project.
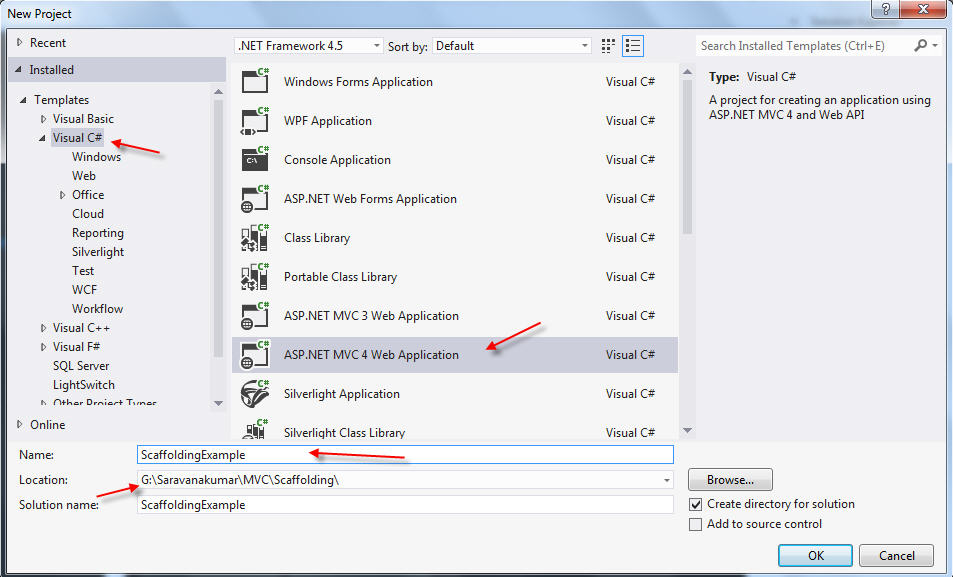
Step 2: Select the Internet project template and click Ok
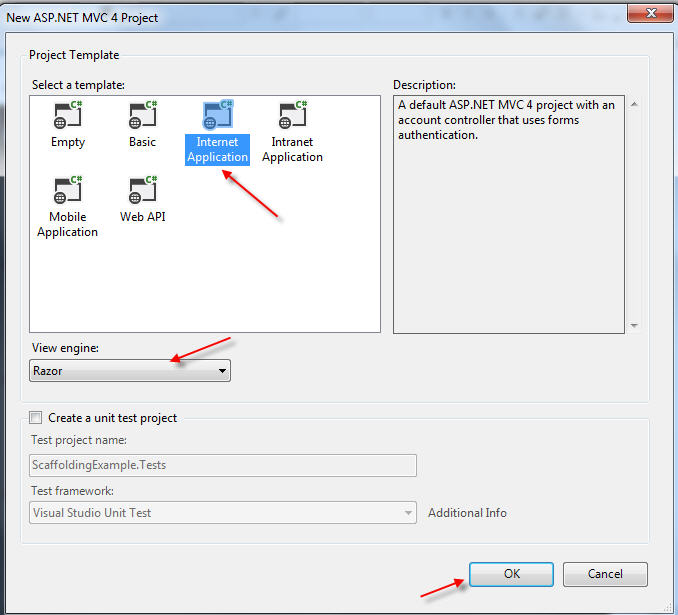
Step 3: Create model class Employee.cs and Address.cs as shown
using System.ComponentModel.DataAnnotations; using System.ComponentModel.DataAnnotations.Schema; namespace ScaffoldingExample.Models { public class Employee { [Key] public string Id { get; set; } public string Name { get; set; } public string Designation { get; set; } public int Salary { get; set; } public Address Address { get; set; } } } public class Address { [Key] public string AddressLine1 { get; set; } public string AddressLine2 { get; set; } public string City { get; set; } public string State { get; set; } public string Country { get; set; } public string Pincode { get; set; } }
Step 4: Create the DBContext class for the Employee details to store the data into DB using Entity framework
public class EmployeeDBContext : DbContext { public EmployeeDBContext() : base("name=EmployeeDBContext") { } public DbSet Employees { get; set; } public DbSet Addresses { get; set; } }
Step 5: Since we are using the Entity framework to save and access the data. System will expect the connection to store and read the data. Entity framework will work in form of ‘Code first’, there is no need to create any DB. When DB context object is getting instantiated, system will create the DB with all entity as table.
Create the connection string in web.config file with Name mention in DBContext class
Step 6: Now add a Scaffolding controller by right-clicking on controller folder in your solution and select Add Controller, A dialogue box appears for setting the controller name and scaffolding options. Set the values as mention below and click Add.
Selection the template option as “Controller with Read/Write Actions and Views, Using Entity Framework”.
This option will create the Controller and View class automatically with create, read, delete operation. More information about the templates is available in the session
Note: If model class are not list in the dropdown, compile the project before adding controller.
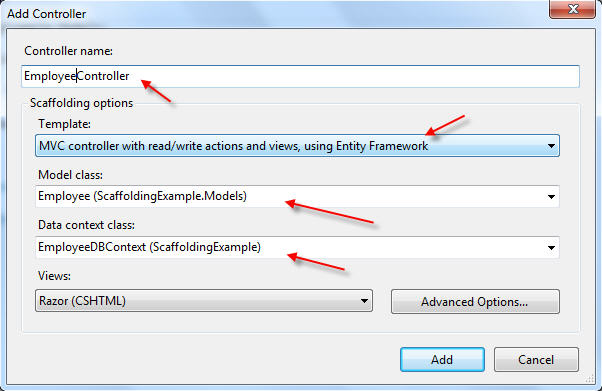
Step 7: Below screen shows the auto generated controller and view files.
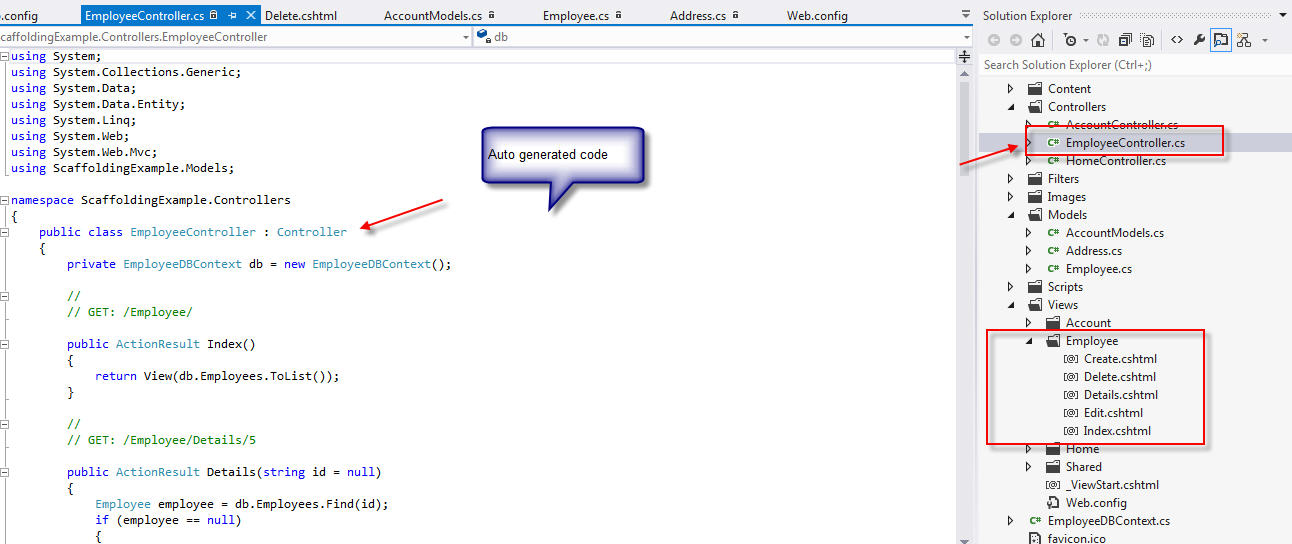
Step(8): Run the application and navigate the Employee “http://localhost:55109/Employee” or “http://localhost:55109/Employee/Create” or “http://localhost:55109/Employee/Create”.
After running the application for first time, database and tables will be created in the SQL server.
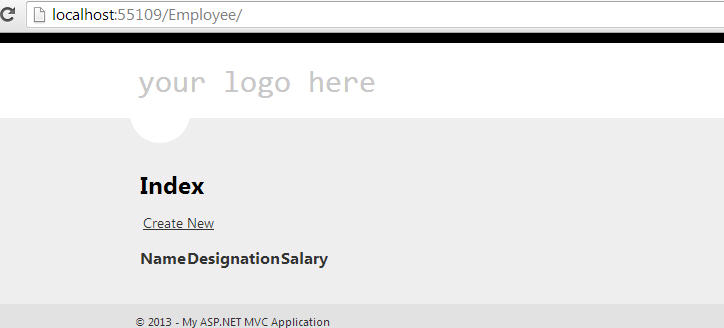
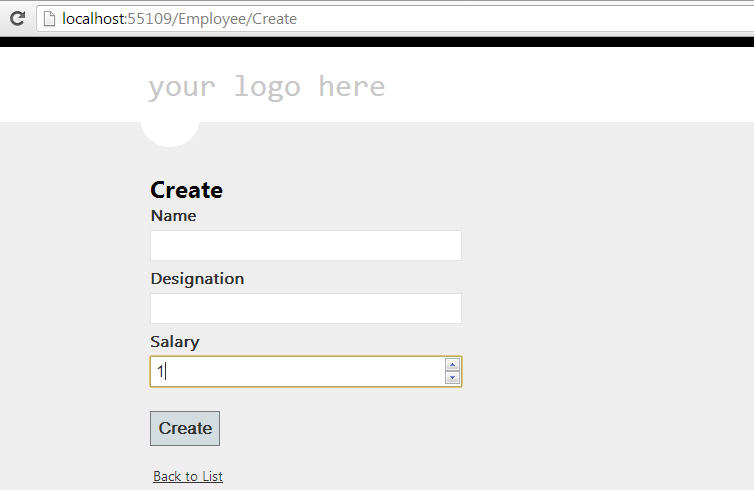