This section is a continuation of the previous section where we created the necessary infrastructure for our Web API.
In this section we will implement Get action methods in our Web API controller class that will handle HTTP GET requests.
As per the Web API naming convention, action method that starts with a work "Get" will handle HTTP GET request. We can either name it only Get or with any suffix. Let's add our first Get action method and give it a name GetAllStudents because it will return all the students from the DB. Following an appropriate naming methodology increases readability and anybody can understand the purpose of a method easily.
The following GetAllStudents() action method in StudentController
class (which we created in the previous section) returns all the students from the database using Entity Framework.
public class StudentController : ApiController
{
public IHttpActionResult GetAllStudents ()
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress")
.Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName
}).ToList<StudentViewModel>();
}
if (students.Count == 0)
{
return NotFound();
}
return Ok(students);
}
}
As you can see in the above example, GetAllStudents() method returns all the students using EF. If no student exists in the DB then it will return 404 NotFound response otherwise it will return 200 OK response with students data. The NotFound()
and Ok()
methods defined in the ApiController returns 404 and 200 response respectively.
In the database, every student has zero or one address. Suppose, you want to implement another GET method to get all the Students with its address then you may create another Get method as shown below.
public class StudentController : ApiController
{
public IHttpActionResult GetAllStudents ()
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress")
.Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName
}).ToList<StudentViewModel>();
}
if (students.Count == 0)
{
return NotFound();
}
return Ok(students);
}
public IHttpActionResult GetAllStudentsWithAddress()
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress").Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName,
Address = s.StudentAddress == null ? null : new AddressViewModel()
{
StudentId = s.StudentAddress.StudentID,
Address1 = s.StudentAddress.Address1,
Address2 = s.StudentAddress.Address2,
City = s.StudentAddress.City,
State = s.StudentAddress.State
}
}).ToList<StudentViewModel>();
}
if (students.Count == 0)
{
return NotFound();
}
return Ok(students);
}
}
The above web API example will compile without an error but when you execute HTTP GET request then it will respond with the following multiple actions found error.
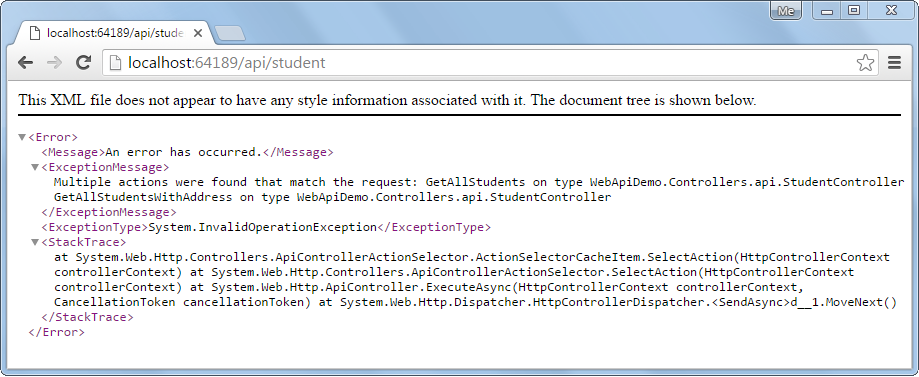
This is because you cannot have multiple action methods with same number of parameters with same type. Both action methods above do not include any parameters. So Web API does not understand which method to execute for the HTTP GET request http://localhost:64189/api/student
.
The following example illustrates how to handle this kind of scenario.
public class StudentController : ApiController
{
public StudentController()
{
}
public IHttpActionResult GetAllStudents(bool includeAddress = false)
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress")
.Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName,
Address = s.StudentAddress == null || includeAddress == false ? null : new AddressViewModel()
{
StudentId = s.StudentAddress.StudentID,
Address1 = s.StudentAddress.Address1,
Address2 = s.StudentAddress.Address2,
City = s.StudentAddress.City,
State = s.StudentAddress.State
}
}).ToList<StudentViewModel>();
}
if (students.Count == 0)
{
return NotFound();
}
return Ok(students);
}
}
As you can see, GetAllStudents action method includes parameter includeAddress with default value false. If an HTTP request contains includeAddress parameter in the query string with value true then it will return all students with its address otherwise it will return students without address.
For example, http://localhost:64189/api/student
(64189 is a port number which can be different in your local) will return all students without address as shown below.
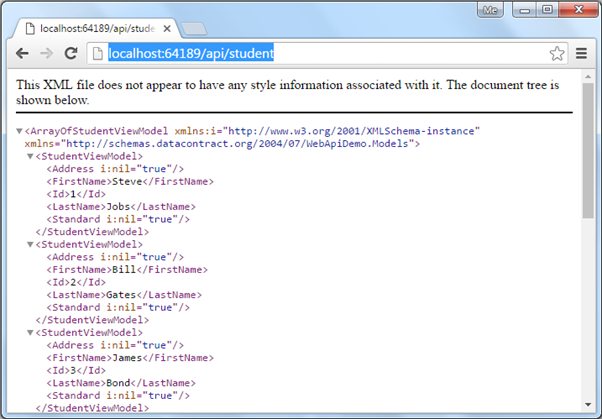
An HTTP request http://localhost:64189/api/student?includeAddress=true
will return all students with address as shown below.

Implement Multiple GET methods:
As mentioned, Web API controller can include multiple Get methods with different parameters and types.
Let's add following action methods in StudentController to demonstrate how Web API handles multiple HTTP GET requests.
Action method | Purpose |
---|---|
GetStudentById(int id) | Returns student whose id matches with the specified id. |
GetAllStudents(string name) | Returns list of students whose name matches with the specified name. |
GetAllStudentsInSameStandard(int standardId) | Returns list of students who are in the specified standard. |
The following example implements the above action methods.
public class StudentController : ApiController
{
public StudentController()
{
}
public IHttpActionResult GetAllStudents(bool includeAddress = false)
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress").Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName,
Address = s.StudentAddress == null || includeAddress == false ? null : new AddressViewModel()
{
StudentId = s.StudentAddress.StudentID,
Address1 = s.StudentAddress.Address1,
Address2 = s.StudentAddress.Address2,
City = s.StudentAddress.City,
State = s.StudentAddress.State
}
}).ToList<StudentViewModel>();
}
if (students == null)
{
return NotFound();
}
return Ok(students);
}
public IHttpActionResult GetStudentById(int id)
{
StudentViewModel student = null;
using (var ctx = new SchoolDBEntities())
{
student = ctx.Students.Include("StudentAddress")
.Where(s => s.StudentID == id)
.Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName
}).FirstOrDefault<StudentViewModel>();
}
if (student == null)
{
return NotFound();
}
return Ok(student);
}
public IHttpActionResult GetAllStudents(string name)
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress")
.Where(s => s.FirstName.ToLower() == name.ToLower())
.Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName,
Address = s.StudentAddress == null ? null : new AddressViewModel()
{
StudentId = s.StudentAddress.StudentID,
Address1 = s.StudentAddress.Address1,
Address2 = s.StudentAddress.Address2,
City = s.StudentAddress.City,
State = s.StudentAddress.State
}
}).ToList<StudentViewModel>();
}
if (students.Count == 0)
{
return NotFound();
}
return Ok(students);
}
public IHttpActionResult GetAllStudentsInSameStandard(int standardId)
{
IList<StudentViewModel> students = null;
using (var ctx = new SchoolDBEntities())
{
students = ctx.Students.Include("StudentAddress").Include("Standard").Where(s => s.StandardId == standardId)
.Select(s => new StudentViewModel()
{
Id = s.StudentID,
FirstName = s.FirstName,
LastName = s.LastName,
Address = s.StudentAddress == null ? null : new AddressViewModel()
{
StudentId = s.StudentAddress.StudentID,
Address1 = s.StudentAddress.Address1,
Address2 = s.StudentAddress.Address2,
City = s.StudentAddress.City,
State = s.StudentAddress.State
},
Standard = new StandardViewModel()
{
StandardId = s.Standard.StandardId,
Name = s.Standard.StandardName
}
}).ToList<StudentViewModel>();
}
if (students.Count == 0)
{
return NotFound();
}
return Ok(students);
}
}
Now, the above Web API will handle following HTTP GET requests.
HTTP GET Request URL | Description |
---|---|
http://localhost:64189/api/student | Returns all students without associated address. |
http://localhost:64189/api/student?includeAddress=false | Returns all students without associated address. |
http://localhost:64189/api/student?includeAddress=true | Returns all students with address. |
http://localhost:64189/api/student?id=123 | Returns student with the specified id. |
http://localhost:64189/api/student?name=steve | Returns all students whose name is steve. |
http://localhost:64189/api/student?standardId=5 | Returns all students who are in 5th standard. |
Similarly you can implement Get methods to handle different HTTP GET requests in the Web API.
The following figure shows HTTP GET request in Fiddler.
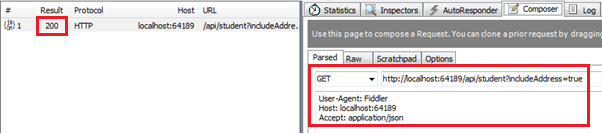
The following figure shows HTTP GET response of above request in Fiddler.
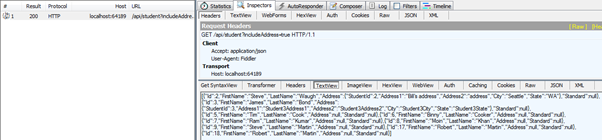
Next, implement Post action method to handle HTTP POST request in the Web API.