Here, you will learn how Web API handles different formats of request and response data.
Media Type:
Media type (aka MIME type) specifies the format of the data as type/subtype e.g. text/html, text/xml, application/json, image/jpeg etc.
In HTTP request, MIME type is specified in the request header using Accept and Content-Type attribute. The Accept header attribute specifies the format of response data which the client expects and the Content-Type header attribute specifies the format of the data in the request body so that receiver can parse it into appropriate format.
For example, if a client wants response data in JSON format then it will send following GET HTTP request with Accept header to the Web API.
GET http://localhost:60464/api/student HTTP/1.1 User-Agent: Fiddler Host: localhost:1234 Accept: application/json
The same way, if a client includes JSON data in the request body to send it to the receiver then it will send following POST HTTP request with Content-Type header with JSON data in the body.
POST http://localhost:60464/api/student?age=15 HTTP/1.1 User-Agent: Fiddler Host: localhost:60464 Content-Type: application/json Content-Length: 13 { id:1, name:'Steve' }
Web API converts request data into CLR object and also serialize CLR object into response data based on Accept and Content-Type headers. Web API includes built-in support for JSON, XML, BSON, and form-urlencoded data. It means it automatically converts request/response data into these formats OOB (out-of the box).
Consider the following simple Post action method.
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
}
public class StudentController : ApiController
{
public Student Post(Student student)
{
// save student into db
var insertedStudent = SaveStudent(student);
return insertedStudent;
}
}
As you can see above, the Post() action method accepts Student type parameter, saves that student into DB and returns inserted student with generated id. The above Web API handles HTTP POST request with JSON or XML data and parses it to a Student object based on Content-Type header value and the same way it converts insertedStudent object into JSON or XML based on Accept header value.
The following figure illustrates HTTP POST request in fiddler.
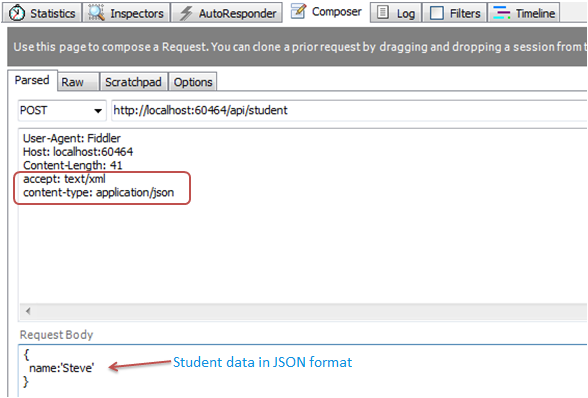
In the above figure, Accept header specifies that it expects response data in XML format and Content-Type specifies that the student data into request body is in the JSON format. The following is the response upon execution of the above request.
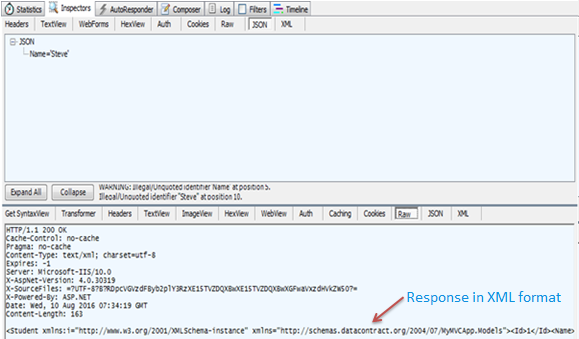
The same way, you can specify different request & response format using accept and content-type headers and Web API will handle them without any additional changes.
The following HTTP POST request sends data in XML format and receives data in JSON format.
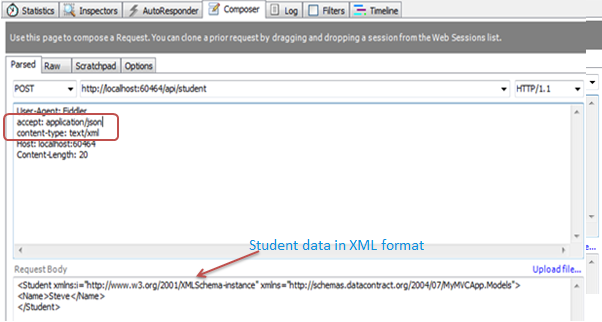
The above HTTP POST request will get the following response upon execution.
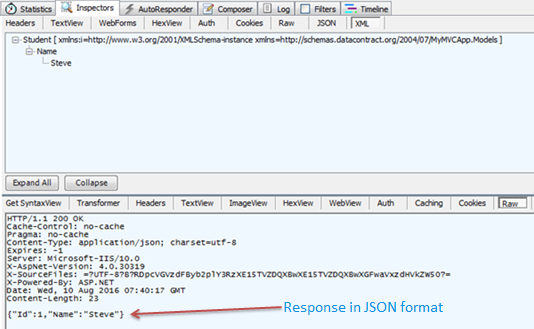
Thus, Web API handles JSON and XML data by default. Learn how Web API formats request/response data using formatters in the next section.