.NET Core Command-line Interface
The .NET Core command-line interface (CLI) is a new cross-platform tool for creating, restoring packages, building, running and publishing .NET applications.
We created our first ASP.NET Core application using Visual Studio in the previous chapter. Visual Studio internally uses this CLI to restore, build and publish an application. Other higher level IDEs, editors and tools can use CLI to support .NET Core applications.
The .NET Core CLI is installed with .NET Core SDK for selected platforms. So we don't need to install it separately on the development machine. We can verify whether the CLI is installed properly by opening command prompt in Windows and writing dotnet and pressing Enter. If it displays usage and help as shown below then it means it is installed properly.
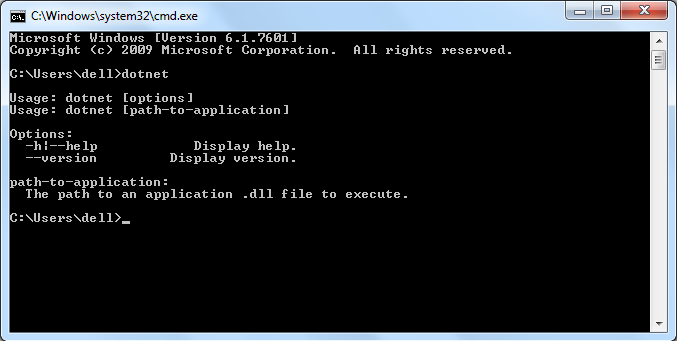
Command Structure:
The following is a command structure.
dotnet <command> <argument> <option>
All the commands start with driver named dotnet. The driver starts the execution of the specified command. After dotnet, we can supply command (also known as verb) to perform a specific action. Each command can be followed by arguments and options. The following are .NET Core 2.x CLI commands.
Basic Commands | Description |
---|---|
new | Creates a new project, configuration file, or solution based on the specified template. |
restore | Restores the dependencies and tools of a project. |
build | Builds a project and all of its dependencies. |
Run | Runs source code without any explicit compile or launch commands. |
publish | Packs the application and its dependencies into a folder for deployment to a hosting system. |
test | Executes unit tests. |
vtest | Runs tests from the specified files. |
pack | Packs the code into a NuGet package. |
clean | Cleans the output of a project. |
sln | Modifies a .NET Core solution file. |
help | Display help on the specified command |
store | Stores the specified assemblies in the runtime package store. |
Project Modification Commands | Description |
---|---|
add package | Adds a package reference to a project. |
add reference | Adds project-to-project (P2P) references. |
remove package | Removes package reference from the project. |
remove reference | Removes project reference |
list reference | Lists all project-to-project references |
Advanced Commands | Description |
---|---|
nuget delete | Deletes or unlists a package from the server. |
nuget locals | Clears or lists local NuGet resources. |
nuget push | Pushes a package to the server and publishes it. |
msbuild | Builds a project and all of its dependencies. |
dotnet install script | Script used to install the .NET Core CLI tools and the shared runtime. |
Let's create, restore, build, and run .NET Core console application using command-line interface without using Visual Studio.
Create a New Project:
To create a new .NET Core project, we have to use new command followed by template name argument. We can create console, class library, web, mvc, webapi, razor, angular, react etc. projects using CLI. Use console template to create a new .NET Core console application.
The following creates new console project in the current directory with the same name as current directory.
dotnet new console
The following command creates a new console project named MyConsoleApp. The -n or --name option species the name of a project.
dotnet new console -n MyConsoleApp
The following command creates a new console application named MyConsoleApp to MyProjects directory. The -o or --output option is used to specify an output directory where the project should be generated.
dotnet new console -n MyConsoleApp -o C:\MyProjects
After creating a project, navigate to the project directories in command prompt to apply project specific commands which is C:\MyConsoleApp in our case.
Add Package Reference:
We often need to add NuGet package reference for different purposes. For example, apply the following command to add Newtonsoft.json package to our console project.
C:\MyConsoleApp>dotnet add package Newtonsoft.json
This will add Newtonsoft.json package to our project. We can verify it by opening .csproj file.
Restore Packages:
To restore packages or to update existing packages, we can use restore command as below.
C:\MyConsoleApp>dotnet restore
Build Project:
To build a new or existing project, apply C:\MyConsoleApp>dotnet build
command.
Run project:
To run our console project, apply dotnet run
command as shown below.
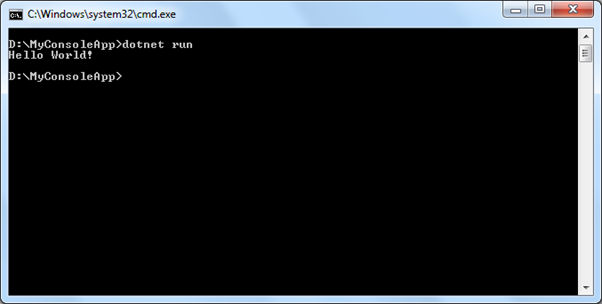
As you can see above, it displays an output "Hello World!".
Getting Help:
We can get help on any .NET Core CLI commands by typing -h or -help at the end of the command we want to get help on. For example, dotnet new -h will display help on the new command, arguments and options we can use with it, as shown below.
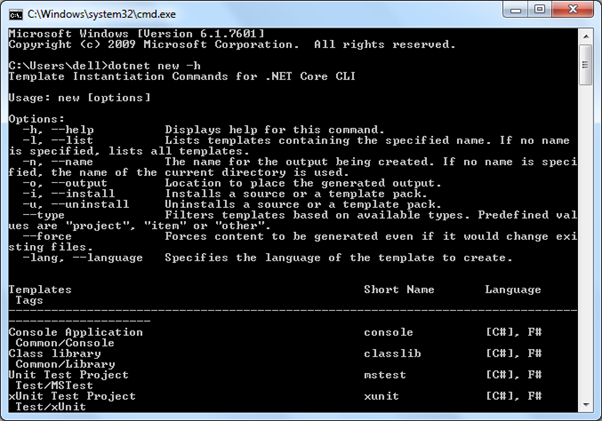
Thus, we can use .NET Core command-line interface to create, restore packages, build, run, and publish different types of .NET Core applications.