C# Data types:
In the previous section, we have seen that a variable must be declared with the data type because C# is a strongly-typed language. For example,
string message = "Hello World!!";
string is a data type, message is a variable, and "Hello World!!" is a string value assigned to a variable - message.
The data type tells a C# compiler what kind of value a variable can hold. C# includes many in-built data types for different kinds of data, e.g., String, number, float, decimal, etc.
class Program
{
static void Main(string[] args)
{
string stringVar = "Hello World!!";
int intVar = 100;
float floatVar = 10.2f;
char charVar = 'A';
bool boolVar = true;
}
}
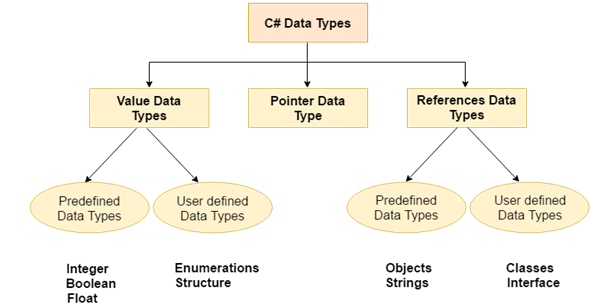
There are 3 types of data types in C# language.
Types | Data Types |
---|---|
Value Data Type | short, int, char, float, double etc |
Reference Data Type | String, Class, Object and Interface |
Pointer Data Type | Pointers |
Each data types includes specific range of values. For example, a variable of int data type can have any value between -2,147,483,648 to 2,147,483,647. The same way, bool data type can have only two value - true or false. The following table lists the data types available in C# along with the range of values possible for each data type:
Alias | .NET Type | Type | Size (bits) | Range (values) |
---|---|---|---|---|
byte | Byte | Unsigned integer | 8 | 0 to 255 |
sbyte | SByte | Signed integer | 8 | -128 to 127 |
int | Int32 | Signed integer | 32 | -2,147,483,648 to 2,147,483,647 |
uint | UInt32 | Unsigned integer | 32 | 0 to 4294967295 |
short | Int16 | Signed integer | 16 | -32,768 to 32,767 |
ushort | UInt16 | Unsigned integer | 16 | 0 to 65,535 |
long | Int64 | Signed integer | 64 | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
ulong | UInt64 | Unsigned integer | 64 | 0 to 18,446,744,073,709,551,615 |
float | Single | Single-precision floating point type | 32 | -3.402823e38 to 3.402823e38 |
double | Double | Double-precision floating point type | 64 | -1.79769313486232e308 to 1.79769313486232e308 |
char | Char | A single Unicode character | 16 | Unicode symbols used in text |
bool | Boolean | Logical Boolean type | 8 | True or False |
object | Object | Base type of all other types | ||
string | String | A sequence of characters | ||
decimal | Decimal | Precise fractional or integral type that can represent decimal numbers with 29 significant digits | 128 | (+ or -)1.0 x 10e-28 to 7.9 x 10e28 |
DateTime | DateTime | Represents date and time | 0:00:00am 1/1/01 to 11:59:59pm 12/31/9999 |
As you can see in the above table that each data types (except string and object) includes value range. Compiler will give an error if value goes out of datatype's permitted range. For example, int data type's range is -2,147,483,648 to 2,147,483,647. So if you assign value which is not in this range then compiler would give error.
// compile time error: Cannot implicitly convert type 'long' to 'int'.
int i = 21474836470;
Alias vs .Net Type:
In the above table of data types, first column is for data type alias and second column is actual .Net type name. For example, int is an alias (or short name) for Int32. Int32 is a structure defined in System namespace. The same way, string represent String class.
Alias | Type Name | .Net Type |
---|---|---|
byte | System.Byte | struct |
sbyte | System.SByte | struct |
int | System.Int32 | struct |
uint | System.UInt32 | struct |
short | System.Int16 | struct |
ushort | System.UInt16 | struct |
long | System.Int64 | struct |
ulong | System.UInt64 | struct |
float | System.Single | struct |
double | System.Double | struct |
char | System.Char | struct |
bool | System.Boolean | struct |
object | System.Object | Class |
string | System.String | Class |
decimal | System.Decimal | struct |
DateTime | System.DateTime | struct |
Data types are further classified as value type or reference type, depending on whether a variable of a particular type stores its own data or a pointer to the data in the memory.
Learn about variables in value type and reference type in the next section.