C# Static:
C# includes "static" keyword just like other programming languages such as C++, Java, etc. The Static keyword can be applied on classes, variables, methods, properties, operators, events and constructors. However, it cannot be used with indexers, destructors or types other than classes.
The Static modifier makes an item non-instantiable, it means the static item cannot be instantiated. If the static modifier is applied to a class then that class cannot be instantiated using the new keyword. If the static modifier is applied to a variable, method or property of class then they can be accessed without creating an object of the class, just use className.propertyName, className.methodName.
public static class MyStaticClass
{
public static int myStaticVariable = 0;
public static void MyStaticMethod()
{
Console.WriteLine("This is a static method.");
}
public static int MyStaticProperty { get; set; }
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine(MyStaticClass.myStaticVariable);
MyStaticClass.MyStaticMethod();
MyStaticClass.MyStaticProperty = 100;
Console.WriteLine(MyStaticClass.MyStaticProperty);
}
}
This is a static method.
100
In the above example, MyStaticClass is a static class with static variable, method and property. All the static members can be access using className without creating an object of a class e.g. MyStaticClass.MyStaticMethod().

It is also possible to have static members in non-static classes just like a normal class. You can instantiate non static classes using the new keyword as usual. However, the instance variable can only access the non-static methods and variables, it cannot access the static methods and variables.
For example, consider the following myNonStaticClass with mix of static and non-static methods:
public class MyNonStaticClass
{
private static int myStaticVariable = 0;
public static void MyStaticMethod()
{
Console.WriteLine("This is static method.");
}
public void myNonStaticMethod()
{
Console.WriteLine("Non-static method");
}
}
In the above example, MyNonStaticClass can be instantiated and access the non-static members. However, you cannot access static members. The following figure shows the debug view.
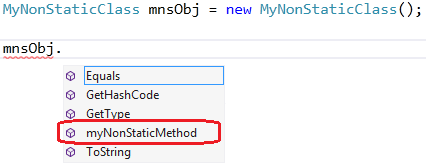
Static Constructor:
A static or non-static class can have a static constructor without any access modifiers like public, private, protected, etc.
A static constructor in a non-static class runs only once when the class is instantiated for the first time.
A static constructor in a static class runs only once when any of its static members accessed for the first time.
public static class MyStaticClass
{
static MyStaticClass()
{
Console.WriteLine("Inside static constructor.");
}
public static int myStaticVariable = 0;
public static void myStaticMethod()
{
Console.WriteLine("This is static method.");
}
public static int MyStaticProperty { get; set; }
}
class Program
{
static void Main(string[] args)
{
MyStaticClass.myStaticVariable = 100;
MyStaticClass.MyStaticProperty = 200;
MyStaticClass.myStaticVariable = 300;
MyStaticClass.MyStaticProperty = 400;
}
}
In the above example, the static members was accessed multiple times. However, static constructor got called only once when any of its static members was accessed for the first time.
public class MyNonStaticClass
{
static MyNonStaticClass()
{
Console.WriteLine("Inside static constructor.");
}
public void myNonStaticMethod()
{
Console.WriteLine("Non-static method");
}
}
class Program
{
static void Main(string[] args)
{
MyNonStaticClass mnsObj1 = new MyNonStaticClass();
MyNonStaticClass mnsObj2 = new MyNonStaticClass();
MyNonStaticClass mnsObj3 = new MyNonStaticClass();
}
}
In the above example, we instantiate MyNonStaticClass three times but the static constructor got called only once when it instantiated for the first time.
Memory allocation of static items:
As you know, the main parts of an application's memory are stack and heap. Static members are stored in a special area inside the heap called High Frequency Heap. Static members of non-static classes are also stored in the heap and shared across all the instances of the class. So the changes done by one instance will be reflected in all the other instances.
As mentioned earlier, a static member can only contain or access other static members, because static members are invoked without creating an instance and so they cannot access non-static members.
The following image illustrates how static members are stored in memory:
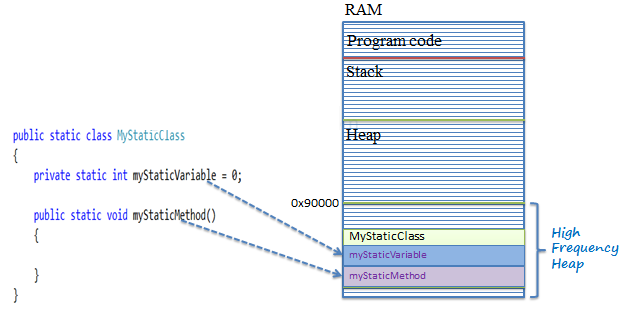

Points to Remember :
- Static classes cannot be instantiated using the new keyword
- Static items can only access other static items. For example, a static class can only contain static members, e.g., variables, methods, etc. A static method can only contain static variables and can only access other static items.
- Static items share the resources between multiple users.
- Static cannot be used with indexers, destructors or types other than classes.
- A static constructor in a non-static class runs only once when the class is instantiated for the first time.
- A static constructor in a static class runs only once when any of its static members accessed for the first time.
- Static members are allocated in high frequency heap area of the memory.
Learn about an anonymous method next