Area:
You have already learned that ASP.NET MVC framework includes separate folders for Model, View and Controller. However, large application can include a large number of controller, views and model classes. So to maintain a large number of views, models and controllers with the default ASP.NET MVC project structure can become unmanageable
ASP.NET MVC 2 introduced Area. Area allows us to partition large application into smaller units where each unit contains separate MVC folder structure, same as default MVC folder structure. For example, large enterprise application may have different modules like admin, finance, HR, marketing etc. So an Area can contain separate MVC folder structure for all these modules as shown below.
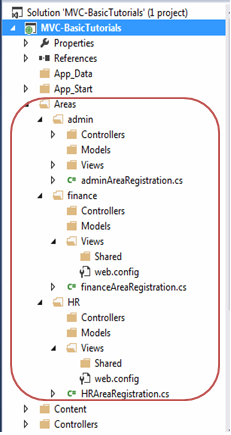
Create Area:
You can create an Area using ASP.NET MVC 5 and Visual Studio 2013 for web by right clicking on the project in the solution explorer -> Add -> Area..
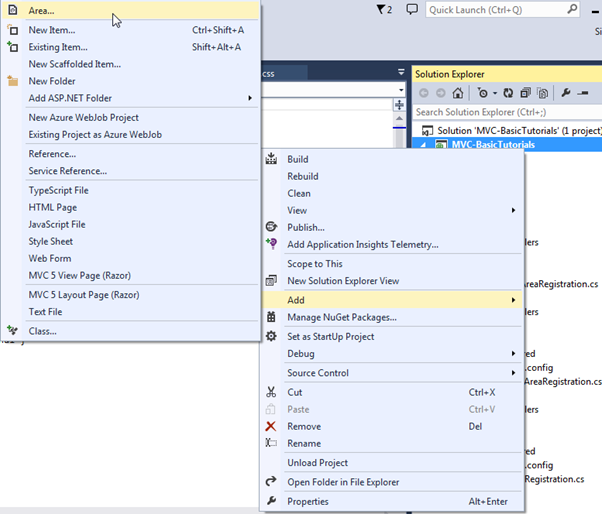
Enter Area name in Add Area dialogue box and click Add.
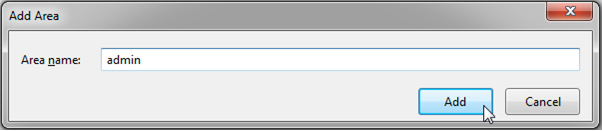
This will add 'admin' folder under Area folder as shown below.
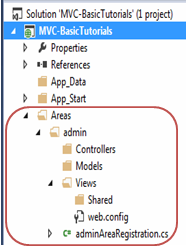
As you can see, each area includes AreaRegistration class in {area name} + AreaRegistration.cs file.
The following is adminAreaRegistration class created with admin area.
public class adminAreaRegistration : AreaRegistration
{
public override string AreaName
{
get
{
return "admin";
}
}
public override void RegisterArea(AreaRegistrationContext context)
{
context.MapRoute(
"admin_default",
"admin/{controller}/{action}/{id}",
new { action = "Index", id = UrlParameter.Optional }
);
}
}
AreaRegistration class overrides RegisterArea method to map the routes for the area. In the above example, any URL that starts with admin will be handled by the controllers included in the admin folder structure under Area folder. For example, http://localhost/admin/profile will be handled by profile controller included in Areas/admin/controller/ProfileController folder.
Finally, all the area must be registered in Application_Start event in Global.asax.cs as AreaRegistration.RegisterAllAreas();
So in this way, you can create and maintain multiple areas for the large application.