Bundling:
Bundling and minification techniques were introduced in MVC 4 to improve request load time. Bundling allow us to load the bunch of static files from the server into one http request.
The following figure illustrates the bundling techniques:
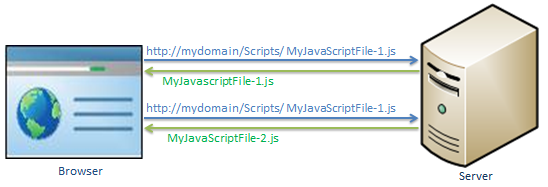
In the above figure, browser sends two separate requests to load two different JavaScript file MyJavaScriptFile-1.js and MyJavaScriptFile-2.js.
Bundling technique in MVC 4 allows us to load more than one JavaScript file, MyJavaScriptFile-1.js and MyJavaScriptFile-2.js in one request as shown below.
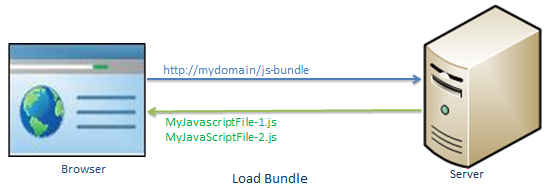
Minification:
Minification technique optimizes script or css file size by removing unnecessary white space and comments and shortening variable names to one character.
For example, consider following JavaScript function.
sayHello = function(name){
//this is comment
var msg = "Hello" + name;
alert(msg);
}
The above JavaScript will be optimized and minimized into following script.
sayHello=function(n){var t="Hello"+n;alert(t)}
As you can see above, it has removed unnecessary white space, comments and also shortening variable names to reduce the characters which in turn will reduce the size of JavaScript file.
Bundling and minification impacts on the loading of the page, it loads page faster by minimizing size of the file and number of requests.
Bundle Types:
MVC 5 includes following bundle classes in System.web.Optimization namespace:
ScriptBundle: ScriptBundle is responsible for JavaScript minification of single or multiple script files.
StyleBundle: StyleBundle is responsible for CSS minification of single or multiple style sheet files.
DynamicFolderBundle: Represents a Bundle object that ASP.NET creates from a folder that contains files of the same type.
All the above bundle classes are included in System.Web.Optimization.Bundle namespace and derived from Bundle class.
Learn about ScriptBundle in the next section.

Points to Remember :
- Bundling and Minification minimize static script or css files loading time therby minimize page loading time.
- MVC framework provides ScriptBundle, StyleBundle and DynamicFolderBundle classes.
- ScriptBundle does minification of JavaScript files.
- StyleBundle does minification of CSS files.
Learn about ScriptBundle next.